Improving website performance
Learn how to analyze and improve the performance of your website
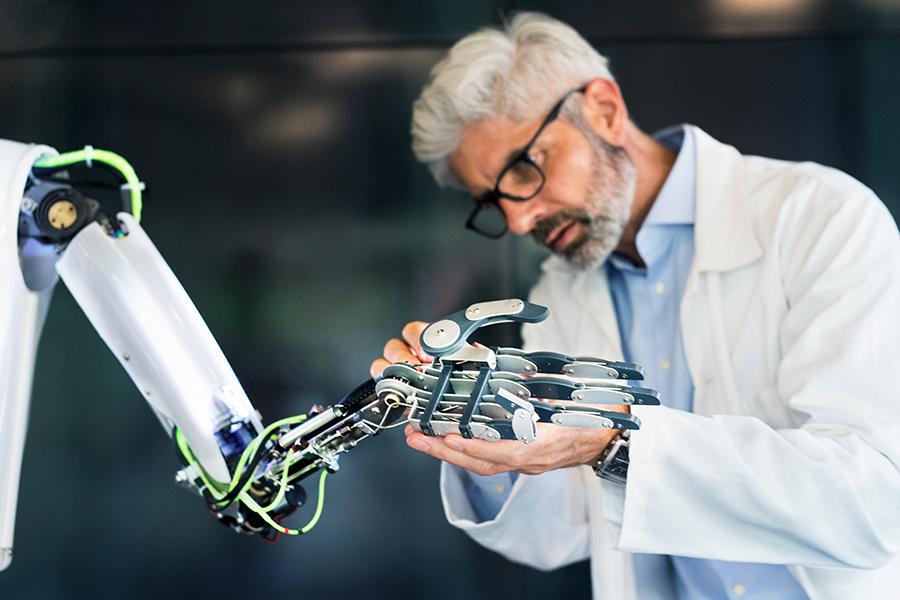
How do we analyze Website Performance?
I) Manual Testing
The first thing to do in order to know if our website is performing at an acceptable standard is to view it from the user’s perspective. We can do this by interacting with the website we have developed. This is a manual way to analyze performance but it can offer important clues.
There are specific things you could ask yourself while doing this manual testing of website performance
- What is the general feel when loading the website for the first time. Is it snappy or is it slow?
- How long does it take to see/load the first paint of the website? (A paint is anything/component that exists on the web page)
- How long does it take for images to load on the a page? Do users actively see images load every time or only the first time?
- If there is content that will take a little bit of time to load, is there a suitable loading indication?
Findings from Manual Testing
There are a lot of takeaways from manual testing. One of the most important observation is the load time of the website and its content: either the website takes a long time to load initially or content on the page takes a lot of time to fetch and display.
II) Automatic Testing
One of the ways to automatically test the performance of a website without is to use the free Chrome development tool Lighthouse.
Lighthouse tells us the performance rating of the website. It also suggests ways in which we can improve the performance. Many of these ways will be touched on in this article.
How do we make sure our website performs well?
Causes of Slow Websites
-
Unoptimized Images
A bunch of unoptimized images is normally a major reason why websites are slow. Images that are high in resolution normally take a lot of time to download. This can also consume the user's bandwidth.
There are image formats which are recommended for websites. Formats such as JPEG and WebP are typically smaller in size than the PNG and GIF counterparts
Recommendation:
- Check the size of an image before using it on your website. Going beyond 1.5MB is too much
- Use formats such as WebP and JPEG
-
HTTP Requests
Do you know that HTTP is what we use to make network requests? Excessive HTTP requests can cause website content load slowdowns
The concept behind this
HTTP runs over a transport layer protocol; most websites use TCP.
When we make a request to a server for an image, there is round-trip time. The round-trip time is the time it takes to send the first packet to the destination (a request for an image), plus the time taken to receive the response packet.
We also have a connection phase with TCP. When the client requests, it receives an acknowledgment from the server. (Handshaking)
Too many HTTP requests can cause website slowdowns because of this.
What we need to do
- Reducing the number of http requests helps.
- Reducing the number of assets that load synchronously. These include CSS, image and JavaScript files.
- Minify your CSS and JavaScript files.
- Get rid of scripts and CSS you dont use on the website but are included in the website.
- If you developed your own server/backend make sure your code is optimized so it responds at a good time.
-
Not making use of Caching
Caching is one of the most important things to do in other to improve performance. Even computers use this technique to improve performance.
The concept is if you have requested an asset before and it typically takes a long time to fetch, why not store it somewhere and reuse it when you need to fetch it again?
Modern-day computers make use of this. When you request a document on your computer, for instance, your computer checks the cache to see if that document is in the cache. If it is in the cache, your computer fetches it from the cache. If it is not in the cache, your computer fetches it from the slower memory and caches it.
Similarly, websites can also use this technique.
By implementing browser and server-side caching, you will see a huge improvement in website performance.
-
Terrible Code
Sometimes it is just your code.
Paying attention to the runtime complexity of critical sections of your code is important.
For example, I would like to get the profile image of a specific
userid
and display it. Let us say I have 40 thousand users.Code Snippet 1 (TypeScript)
Runtime complexity - O(n)
let listOfUserObjects: User[] = [40 million entries]; getProfileImage(uid: string) : string { listOfUserObjects.forEach(user => { if (user.uid.equals(uid)){ return user.image; } }); return "No Image"; }
Code Snippet 2 (TypeScript)
Runtime complexity - O(1)
let map = new Map(); // Map consiting of userId -> profileimage getProfileImage(uid: string) : string { return map.get(uid); }
Looking at both of these code snippets, we can see that the code in snippet 1 is much slower. Going through a list of 40 thousand entries would mean that the profile image will load, on averge, much later than if loaded via snippet 2.
For snippet 2, a map has runtime complexity of O(1) and means the profile image would be retrieved almost instantly.
-
Assets
When developing your website, having unnecessary white space, inline styles and comments make the stylesheet larger in size hence, it takes more time to download. Try to make your CSS file sizes as little as possible and try to minify them.
-
Lazy loading
Lazy loading is the concept of not loading an object or asset until it is needed. Sometimes, there is no need to load all the assets on a website.
For example, loading all the images in a list of 500 images at the initial load time will slow down the website load speed. A better way to do it would be to wait until the user needs it. When the user scrolls down to an image that is not loaded, the image can load or the image and the next 5 images.
-
Using CDN's
A CDN (Content Delivery Network) is a network of servers deployed in several geographic locations, that serve content to visitors.
Depending on where the user is located, requested content will be served by the server closest to the user. This minimizes the round-trip time we discussed in the 'HTTP Requests' section above.
Conclusion
There are a variety of techniques to improve your website performance and load speed. The most important things to remember are: Terrible Code, HTTP Requests, Unoptimized Images, Lazy loading, and Caching.
Sometimes it is difficult figuring out what causes a performance dip but using a combination of the manual testing techniques and automatic testing techniques in this article, you will be able to know what is causing a performance issue.